Web APIs - Using Vendor Management APIs
Introduction
In the case where a TI has:
- A web based solution
- A multi school solution
- More than a handful of schools using the application
Then the use of the vendor management APIs offer a simple and effective way to process perhaps data from 1000s of schools without the need to manually manage the credentials.
How is this done?
Vendor Management Tile in SIMS ID
You will need your TI SIMS ID login. If this is an issue contact partner support.
Go in to Technical Integrators from the home page and a new tile is available
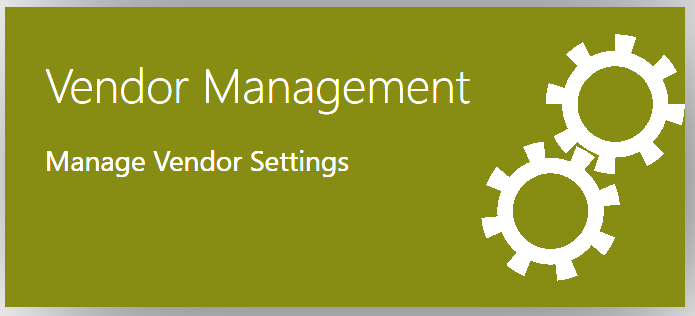
Click on the tile and you can:
a. Claim vendor management credentials
b. Collect vendor management credentials
c. Reset the client secret where required.
Warning - these credentials allow the harvesting of credentials for all of your customers. They must be stored in a secure place such as Key Vault. Example keys shown are limited to demo systems and test data.
What can these credentials achieve?
Use the Token to get:
a. A list of schools using a TI's application identified by an application ID.
b. For each of the schools choosing that application in the tile store, we can access the credentials.
Sample Code
Key data classes
public class Customer
{
public int? Id;
public string Name;
public Guid? OrganisationId;
public string DfeCode;
public string DeniNumber;
public int? Authorised;
public Credential Credentials;
}
public class Credential
{
public string ClientId;
public string Secret;
public string OCPAIMKey;
public string Description;
}
Get a token for the Vendor management APIs - Vendor Management JWT (JSOn Web Token)
/// <summary>
/// Get a Vendor Management token which allows the TI to return the schoosl using the requested app
/// and subsequently enables requests to get the credentials for the APIs to get data from the school's system.
/// </summary>
/// <returns></returns>
public static string VendorManagementToken()
{
AuthRequest arq = new AuthRequest();
string rc = "";
// warning - IF THESE KEYS AND SECRETS ARE LOST THEN A MALICIOUS ACTOR COULD ACCESS
// DATA IN ALL OF YOUR SCHOOLS
// We recommend Key Vault or similar to store these!
arq.Client_ID = "vmc-c855a013-b7f1-4754-b5be-1b00ea03afc0";
arq.Secret = "r6KyftsCSc3Vt5MF";
// WARNING
arq.Organisation_ID = null;
arq.STS = "https://sts.sims.co.uk/connect/token";
// Note the different scopes.
arq.Scopes = "partner-read partner-write";
BearerToken bt = new BearerToken(arq, httpClient);
rc = bt.Token;
return rc;
}
Flow of the application
/// <summary>
/// API Manager key is needed to access DeX endpoints - a sample value is below which will be throttled.
/// </summary>
public static string OCP_Key = "e3160e947ac44fa4a4135a3e2bd29c1e";
/// <summary>
///
/// This can be accessed via the SIMS UI - Edit the application in SIMS ID
/// Look at the URL
/// https://ti.sims.co.uk/Applications/ManageApplication?applicationId=2585d852-956b-457d-b510-03584a3f02bf&siteId=18996
/// and extract the applicationId like in the example above.
///
/// </summary>
public static string ApplicationID = "2585d852-956b-457d-b510-03584a3f02bf";
public static CallInfo callInfo = new CallInfo();
public static HttpClient httpClient = new HttpClient();
static void Main(string[] args)
{
// Get the vendo management token
string token = VendorManagementToken();
// Use it to get a list of schools using the requested app.
Call c = new Call(string.Format("https://rap.sims.co.uk/api/v1/partner/{0}/GetSiteByApplication", ApplicationID));
string resp = c.Execute(token, httpClient, "");
// Create a list of customers (schools)
var _Customers = Newtonsoft.Json.JsonConvert.DeserializeObject<List<Customer>>(resp);
// for each school - see what credentials are available.
This sets up and makes the call to get the list of customers using the application, then for each customer we can get one or more School Data Access JWT from SIMS ID to enable the application to get that school's data.
Please don't confuse the Vendor Management JWT with the School Data Access JWT, whilst they are requested from the same token server, they serve very different purposes.
We can then use the credential data returned to:
a. Get an School Data Access JWT
b. Request data from the appropriate system
// For each of these get some data
foreach (Credential cred in _Credential)
{
if (cred.ClientId.Contains("oneroster"))
{
// One roster scopes are different to OData DeX!
string dataToken = SchoolDataAccessToken(cred.ClientId, cred.Secret, "onerosterapi rapapi", cus.OrganisationId.ToString());
Call c3 = new Call("https://simsid-partner-oneroster.azurewebsites.net/ims/oneroster/v1p1/schools/");
string resp3 = c3.Execute(dataToken, httpClient, "");
// Get some data from one roster - even an empty set is success
}
else
{
// Use the DeX scopes to get some data.
string dataToken = SchoolDataAccessToken(cred.ClientId, cred.Secret, "partnerserverapplication partner", cus.OrganisationId.ToString());
Call c3 = new Call("https://seli00apm01.azure-api.net/dex/Learner/odata/v3/LearnerIdentifiers");
string resp3 = c3.Execute(dataToken, httpClient, OCP_Key);
}
}
Please note that applications may have multiple credentials and each credential needs to be identified and the correct scopes used to get the required data. Alternatively if data is only needed from a single service then only one set of credentials would be returned.
/// <summary>
/// Gets a token for RAP / One Roster or DeX -
///
/// </summary>
/// <param name="clientId"></param>
/// <param name="secret"></param>
/// <param name="scopes">Scopes need to reflect the call requested.</param>
/// <param name="orgId"></param>
/// <returns></returns>
public static string SchoolDataAccessToken(string clientId, string secret,string scopes, string orgId)
{
AuthRequest arq = new AuthRequest();
string rc = "";
arq.Client_ID = clientId;
arq.Secret = secret;
arq.Organisation_ID = orgId; // Must have one
arq.STS = "https://sts.sims.co.uk/connect/token";
arq.Scopes = scopes;
BearerToken bt = new BearerToken(arq, httpClient);
rc = bt.Token;
return rc;
}
Download
Sample code is available on this link.